- A+
所属分类:阁主小札
Dart版本
/*DynamicAuth 动态加密 authcode({"d":data,"t":timeout,"s":md5}) md5 = md5(d-md5key-t) */ class DynamicAuth { static const int Ok = 0; static const int ErrTimeout = 1; static const int ErrMd5CheckFailed = 2; static const int ErrFormat = 3; static const int ErrAuthcode = 4; String key; String md5key; Duration dur; DynamicAuth( {String key = "", String md5key = "", Duration dur = const Duration(seconds: 20)}) { this.key = key; this.md5key = md5key; this.dur = dur; } String encode(String str) { MapjsonMap = new Map (); jsonMap["d"] = str; DateTime curTime = DateTime.now().add(dur); jsonMap["t"] = (curTime.millisecondsSinceEpoch ~/ 1000).toString(); jsonMap["s"] = hex_md5(str + "-" + this.md5key + "-" + jsonMap["t"]); return authcode(json.encode(jsonMap), "ENCODE", this.key); } Map decode(String str) { Map res = new Map (); //authcode String authcoded = authcode(str, "DECODE", this.key); if (authcoded == "") { res["ret"] = ErrAuthcode; return res; } //json_decode Map jsonDecoded; try { jsonDecoded = json.decode(authcoded); } catch (e) { res["ret"] = ErrFormat; res["data"] = e.toString(); return res; } if (jsonDecoded["d"] is! String || jsonDecoded["t"] is! String || jsonDecoded["s"] is! String) { res["ret"] = ErrFormat; res["data"] = "Missing element or type is wrong"; return res; } //md5 check if (hex_md5( jsonDecoded["d"] + "-" + this.md5key + "-" + jsonDecoded["t"]) != jsonDecoded["s"]) { res["ret"] = ErrMd5CheckFailed; return res; } //time check int curTimestamp = (DateTime.now().millisecondsSinceEpoch) ~/ 1000; int timeout; try { timeout = int.parse(jsonDecoded["t"]); } catch (e) { res["ret"] = ErrFormat; res["data"] = e.toString(); return res; } if (curTimestamp > timeout) { res["ret"] = ErrTimeout; return res; } //ok res["ret"] = Ok; res["data"] = jsonDecoded["d"]; return res; } }
Go版本
const ( //AuthOk 解密成功 AuthOk int = iota //ErrTimeout 超时 ErrTimeout //ErrFormat 格式错误 ErrFormat //ErrMd5CheckFailed md5校检错误 ErrMd5CheckFailed //ErrAuthcode Authcode错误 ErrAuthcode ) /*NewDynamicAuth 新建动态加密 authcode({"d":data,"t":timeout,"s":md5}) md5 = md5(d-md5key-t) */ func NewDynamicAuth(key, md5key string, dur time.Duration) *DynamicAuth { da := new(DynamicAuth) da.Key = key da.Md5Key = md5key da.Dur = dur return da } //DynamicAuth 动态加密 type DynamicAuth struct { Key string Md5Key string Dur time.Duration } //Encode 加密 func (c *DynamicAuth) Encode(str string) string { jsonMap := make(map[string]string) jsonMap["d"] = str jsonMap["t"] = strconv.FormatInt(time.Now().Add(c.Dur).Unix(), 10) jsonMap["s"] = HexMd5(str + "-" + c.Md5Key + "-" + jsonMap["t"]) bs, err := json.Marshal(&jsonMap) if err != nil { fmt.Println(err) return "" } jsonEncoded := string(bs) return Authcode(jsonEncoded, "ENCODE", c.Key) } //Decode 解密 func (c *DynamicAuth) Decode(str string) (string, int) { var ( err error timeout int64 jsonDecoded map[string]string ) //authcode authcoded := Authcode(str, "DECODE", c.Key) if authcoded == "" { return "", ErrAuthcode } //json_decode err = json.Unmarshal([]byte(authcoded), &jsonDecoded) if err != nil { return err.Error(), ErrFormat } if jsonDecoded["d"] == "" || jsonDecoded["t"] == "" || jsonDecoded["s"] == "" { return "Missing element", ErrFormat } //md5 check if HexMd5(jsonDecoded["d"]+"-"+c.Md5Key+"-"+jsonDecoded["t"]) != jsonDecoded["s"] { return "", ErrMd5CheckFailed } //time check timeout, err = strconv.ParseInt(jsonDecoded["t"], 10, 64) if err != nil { return err.Error(), ErrFormat } if (time.Now().Unix()) > timeout { return "", ErrTimeout } //ok return jsonDecoded["d"], AuthOk }
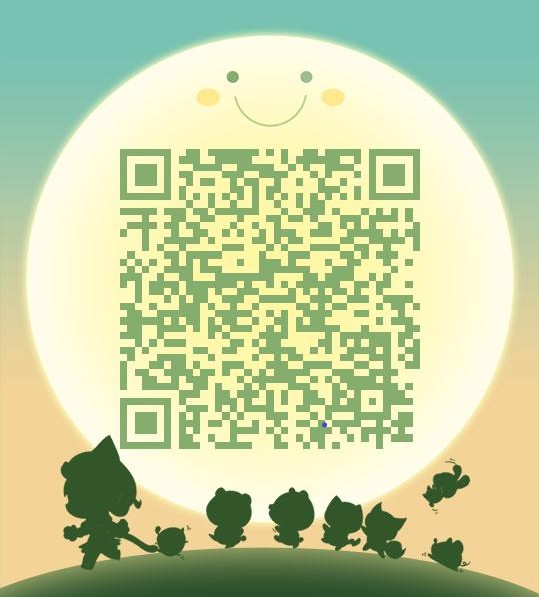
画麟阁QQ群
这是一个有爱的大家庭,也是东阁唯一的社区,快来和大家一起闲聊、讨论吧!